Pattern matching is not merely about recognizing data structures; it's also a powerful tool for deconstructing and extracting data. You can use patterns to bind variables to specific parts of the data, making it accessible for further processing.Pattern matching is the technique that identifies specific patterns or sequences or a combination of characters within a larger piece of information. Take an example of searching for a specific word in the book. You can use pattern matching to find all instances of that word present in the book.Boyer-Moore algorithm (also recognized as BM) (Boyer and Moore, 1977) is known to be very fast in practice. It performs character comparisons between a character in the text and a character in the pattern database from right to left.
What is the pattern matching problem : Pattern matching aims at design of algorithms that can efficiently look for similar pattern across large sequences. Identification of patterns (also referred to as signatures), for generating interaction maps or expression profiles, is usually an NP-complete problem.
How would I perform pattern matching in C++
Pattern Matching in C++
Use the std::regex class to create a regular expression object, passing the pattern as a parameter to the constructor.
Use std::regex_search or std::regex_match to search for or match the pattern within the string.
If a match is found print pattern matched.
What is the best algorithm for pattern matching : One of the most popular Pattern Matching algorithms is the Boyer-Moore algorithm. This algorithm was first published in 1977 by Robert S. Boyer and J Strother Moore.
One of the best examples of an efficient algorithm is the Binary Search. With a Big O notation of O(log n), it's used to search for a specific element in a sorted list. Binary Search repeatedly divides the search interval into two equal halves until it finds the target element. The match_results::operator[] is a built function in C++ which used to get the i-th match in the match_result object. It gives the reference to the match at the position given inside the operator.
Are patterns important in C++
Design patterns are tried-and-tested solutions to common software design problems. They are not specific to a programming language but can be applied to different languages, including C++. Design patterns help you create code that is more modular, flexible, and easier to maintain.(but is slow in Java, Perl, PHP, Python, Ruby, …)Bogosort is generally considered to be the worst sorting algorithm out there. Other names for it include Monkey Sort and Random Sort. The idea behind Bogosort comes from the field of probability theory, which asserts that if anything is conceivable, it will happen eventually. Here are 5 of those algorithms.
Euclid's Algorithm. Amateur Approach.
Depth First Tree Traversal. Let's write a Binary tree to begin with.
Sieve of Eratosthenes. Amateur Approach.
Calculating Factorials. The iterative approach to finding factorials works as follows.
Generating Fibonacci Numbers.
How to pattern match in C++ : Pattern Matching in C++
Use the std::regex class to create a regular expression object, passing the pattern as a parameter to the constructor.
Use std::regex_search or std::regex_match to search for or match the pattern within the string.
If a match is found print pattern matched.
Can you do regex in C++ : The C++ standard library supports multiple regular expression grammars.
How would perform pattern matching in C++
Pattern Matching in C++
Use the std::regex class to create a regular expression object, passing the pattern as a parameter to the constructor.
Use std::regex_search or std::regex_match to search for or match the pattern within the string.
If a match is found print pattern matched.
The logic is as follows :
Take input from the user of the number of rows in the Butterfly pattern.
Iterate from i=0 to i=rows-1 and do the following –
Iterate from j=0 to j=i and print + Blank space i.e.i.e. cout << "" << " ";
Define a variable to find the number of spaces needed to be printed; say it to be spacespace.
contains(String) is much faster than explicitly using Regex .
Why is regex so complicated : Overloaded syntax and context
Regular expressions use a small set of symbols, and so some of these symbols to double duty. For example, symbols take on different meanings inside and outside of character classes. (See point #4 here.) Extensions to the basic syntax are worse.
Antwort Why ++ is not allowed in pattern matching? Weitere Antworten – What are the advantages of pattern matching
Using patterns to destructure and extract data
Pattern matching is not merely about recognizing data structures; it's also a powerful tool for deconstructing and extracting data. You can use patterns to bind variables to specific parts of the data, making it accessible for further processing.Pattern matching is the technique that identifies specific patterns or sequences or a combination of characters within a larger piece of information. Take an example of searching for a specific word in the book. You can use pattern matching to find all instances of that word present in the book.Boyer-Moore algorithm (also recognized as BM) (Boyer and Moore, 1977) is known to be very fast in practice. It performs character comparisons between a character in the text and a character in the pattern database from right to left.

What is the pattern matching problem : Pattern matching aims at design of algorithms that can efficiently look for similar pattern across large sequences. Identification of patterns (also referred to as signatures), for generating interaction maps or expression profiles, is usually an NP-complete problem.
How would I perform pattern matching in C++
Pattern Matching in C++
What is the best algorithm for pattern matching : One of the most popular Pattern Matching algorithms is the Boyer-Moore algorithm. This algorithm was first published in 1977 by Robert S. Boyer and J Strother Moore.
One of the best examples of an efficient algorithm is the Binary Search. With a Big O notation of O(log n), it's used to search for a specific element in a sorted list. Binary Search repeatedly divides the search interval into two equal halves until it finds the target element.
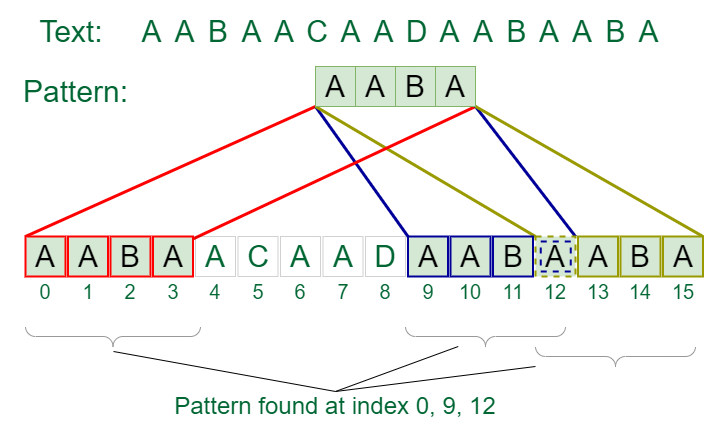
The match_results::operator[] is a built function in C++ which used to get the i-th match in the match_result object. It gives the reference to the match at the position given inside the operator.
Are patterns important in C++
Design patterns are tried-and-tested solutions to common software design problems. They are not specific to a programming language but can be applied to different languages, including C++. Design patterns help you create code that is more modular, flexible, and easier to maintain.(but is slow in Java, Perl, PHP, Python, Ruby, …)Bogosort is generally considered to be the worst sorting algorithm out there. Other names for it include Monkey Sort and Random Sort. The idea behind Bogosort comes from the field of probability theory, which asserts that if anything is conceivable, it will happen eventually.
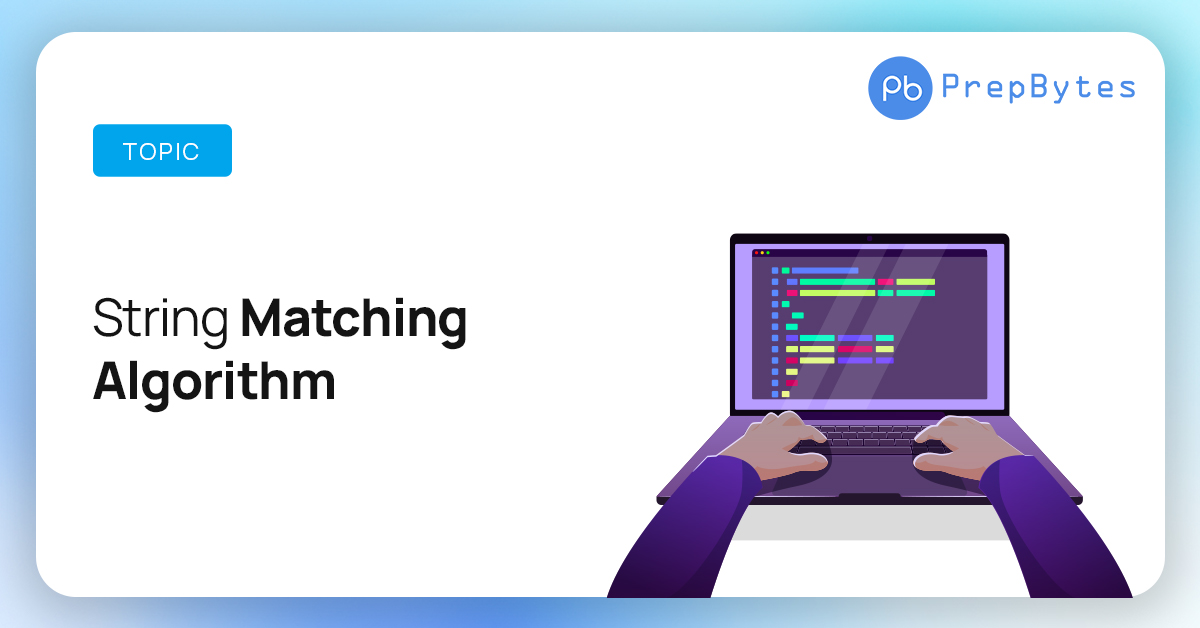
Here are 5 of those algorithms.
How to pattern match in C++ : Pattern Matching in C++
Can you do regex in C++ : The C++ standard library supports multiple regular expression grammars.
How would perform pattern matching in C++
Pattern Matching in C++
The logic is as follows :
contains(String) is much faster than explicitly using Regex .
Why is regex so complicated : Overloaded syntax and context
Regular expressions use a small set of symbols, and so some of these symbols to double duty. For example, symbols take on different meanings inside and outside of character classes. (See point #4 here.) Extensions to the basic syntax are worse.