HashSet is an unordered collection containing unique elements. It has the standard collection operations Add, Remove and Contains, but since it uses a hash-based implementation, these operations are O(1). (As opposed to List for example, which is O(n) for Contains and Remove.)C# – List<T>
List<T> equivalent of the ArrayList, which implements IList<T>.
It comes under System.
List<T> can contain elements of the specified type.
Elements can be added using the Add() , AddRange() methods or collection-initializer syntax.
Elements can be accessed by passing an index e.g. myList[0] .
They have different semantics. A list is ordered (by insert order), allows duplicates, and offers random-access by index; a hash-set is unordered, does not allow duplicates (removes them, by design), and does not offer random-access. Both are perfectly valid, simply: for different scenarios.
Is HashSet faster than list in C# : As expected, Dictionaries and HashSet perform definitely better than List. It's easy to see that in almost all the cases makes no difference which data structure is used if the dataset is relatively small, less than 10000 items.
Why do we use lists in C#
They do provide a dynamic, resizeable, and strongly-typed alternative to arrays. But, what makes Lists so important in C# Dynamic Size – Lists in C# have a dynamic size, meaning that you can add or remove elements as needed without having to worry about the size of the list.
When to use array vs list : If we require fixed length and static allocation then, arrays are used over lists. When the faster processing of data is required then arrays are used over lists. Primitive data types can be stored in arrays directly but not in lists so, we use arrays over lists.
Test Results. The List type is the clear winner here, and no surprise really given that both HashSet and Dictionary ensures that that are no duplicated, what's surprising though is how much more overhead you incur when dealing with reference types! They say hash lookups are fast and it's no lie! Duplicates : ArrayList allows duplicate values while HashSet doesn't allow duplicates values. Ordering : ArrayList maintains the order of the object in which they are inserted while HashSet is an unordered collection and doesn't maintain any order.
Why use list instead of array C#
Lists in C# are like dynamic collections or, in simpler terms, a cool band of elements clutching onto the same data type. Unlike our good old arrays, these step up the game by allowing adding or removing of elements during runtime!Choosing between Lists and Arrays in C# game development depends on your specific requirements. Lists offer flexibility and ease of use, making them a solid choice for dynamic collections. Arrays provide predictable memory usage and quick access, making them suitable for fixed-size collections.Arrays need to be initialized with values or set to default values, while lists can be created and used immediately. Performance: Arrays generally have better performance than lists when it comes to accessing individual elements, but lists are faster when it comes to adding or removing elements from the collection. As expected, Dictionaries and HashSet perform definitely better than List. It's easy to see that in almost all the cases makes no difference which data structure is used if the dataset is relatively small, less than 10000 items.
Which is faster, HashSet or List in C# : Yes a linear search of a List will beat a HashSet for a small number of items. But the performance difference usually doesn't matter for collections that small. It's generally the large collections you have to worry about, and that's where you think in terms of Big-O.
Is HashSet better than list : In a nutshell, HashSet and List are both invaluable tools for managing your data collections, but they excel in different domains. Choose HashSet when uniqueness and efficient membership checks are paramount. Opt for List when you need to maintain elements in a specific order, and duplicates are welcome guests.
What are the disadvantages of list in C#
However, lists also have some disadvantages: Memory inefficiency: Lists require more memory than arrays because they have to store additional information, such as the size of the list and a reference to the next element. This can be a problem if you are working with very large lists. as you can see Array performance is better than 2 others, and List with out size or capacity has lowest performance. Contiguous Memory Allocation : Contiguous memory allocation is basically a method in which a single contiguous section/part of memory is allocated to a process or file needing it.They do provide a dynamic, resizeable, and strongly-typed alternative to arrays. But, what makes Lists so important in C# Dynamic Size – Lists in C# have a dynamic size, meaning that you can add or remove elements as needed without having to worry about the size of the list.
Is list slower than array in C# : Relatively slower individual element access: Accessing individual elements in the List can be slower compared to an Array. It's not they're turtles, but Arrays are just cheetahs.
Antwort What is the difference between hash and list C#? Weitere Antworten – What is the difference between HashSet and list in C#
HashSet is an unordered collection containing unique elements. It has the standard collection operations Add, Remove and Contains, but since it uses a hash-based implementation, these operations are O(1). (As opposed to List for example, which is O(n) for Contains and Remove.)C# – List<T>
They have different semantics. A list is ordered (by insert order), allows duplicates, and offers random-access by index; a hash-set is unordered, does not allow duplicates (removes them, by design), and does not offer random-access. Both are perfectly valid, simply: for different scenarios.

Is HashSet faster than list in C# : As expected, Dictionaries and HashSet perform definitely better than List. It's easy to see that in almost all the cases makes no difference which data structure is used if the dataset is relatively small, less than 10000 items.
Why do we use lists in C#
They do provide a dynamic, resizeable, and strongly-typed alternative to arrays. But, what makes Lists so important in C# Dynamic Size – Lists in C# have a dynamic size, meaning that you can add or remove elements as needed without having to worry about the size of the list.
When to use array vs list : If we require fixed length and static allocation then, arrays are used over lists. When the faster processing of data is required then arrays are used over lists. Primitive data types can be stored in arrays directly but not in lists so, we use arrays over lists.
Test Results. The List type is the clear winner here, and no surprise really given that both HashSet and Dictionary ensures that that are no duplicated, what's surprising though is how much more overhead you incur when dealing with reference types! They say hash lookups are fast and it's no lie!
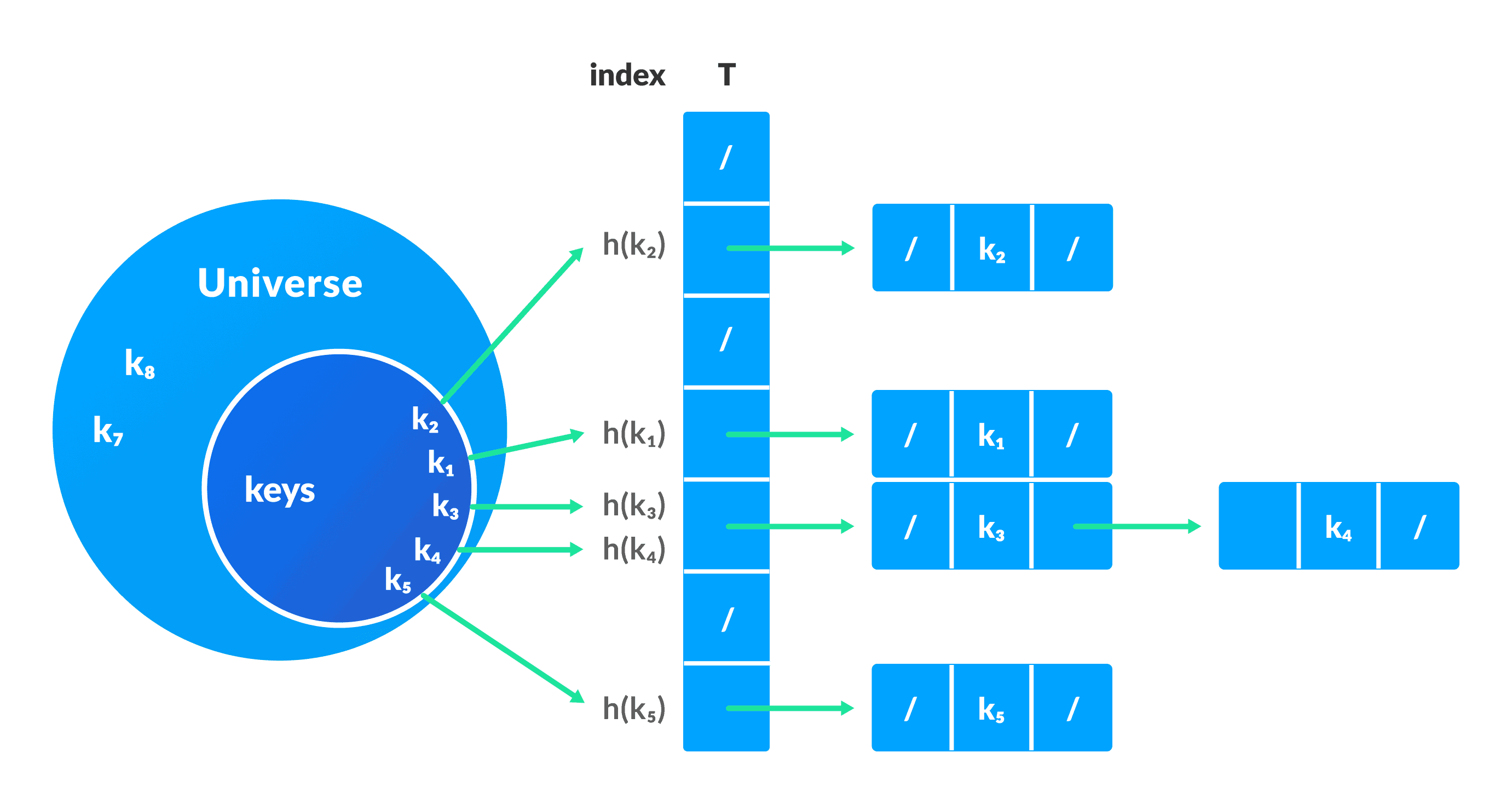
Duplicates : ArrayList allows duplicate values while HashSet doesn't allow duplicates values. Ordering : ArrayList maintains the order of the object in which they are inserted while HashSet is an unordered collection and doesn't maintain any order.
Why use list instead of array C#
Lists in C# are like dynamic collections or, in simpler terms, a cool band of elements clutching onto the same data type. Unlike our good old arrays, these step up the game by allowing adding or removing of elements during runtime!Choosing between Lists and Arrays in C# game development depends on your specific requirements. Lists offer flexibility and ease of use, making them a solid choice for dynamic collections. Arrays provide predictable memory usage and quick access, making them suitable for fixed-size collections.Arrays need to be initialized with values or set to default values, while lists can be created and used immediately. Performance: Arrays generally have better performance than lists when it comes to accessing individual elements, but lists are faster when it comes to adding or removing elements from the collection.
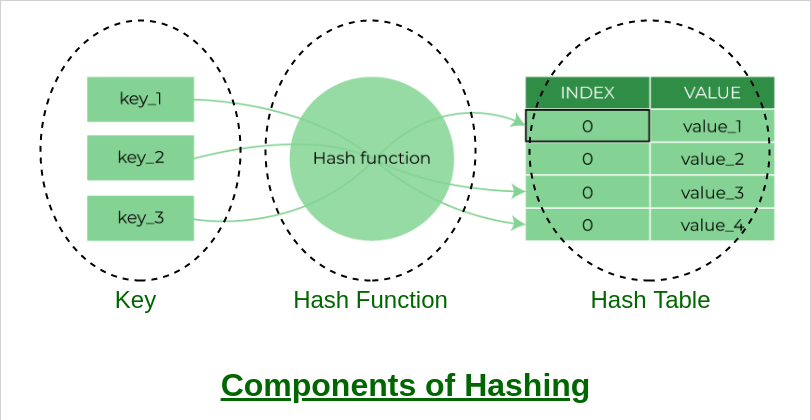
As expected, Dictionaries and HashSet perform definitely better than List. It's easy to see that in almost all the cases makes no difference which data structure is used if the dataset is relatively small, less than 10000 items.
Which is faster, HashSet or List in C# : Yes a linear search of a List will beat a HashSet for a small number of items. But the performance difference usually doesn't matter for collections that small. It's generally the large collections you have to worry about, and that's where you think in terms of Big-O.
Is HashSet better than list : In a nutshell, HashSet and List are both invaluable tools for managing your data collections, but they excel in different domains. Choose HashSet when uniqueness and efficient membership checks are paramount. Opt for List when you need to maintain elements in a specific order, and duplicates are welcome guests.
What are the disadvantages of list in C#
However, lists also have some disadvantages: Memory inefficiency: Lists require more memory than arrays because they have to store additional information, such as the size of the list and a reference to the next element. This can be a problem if you are working with very large lists.

as you can see Array performance is better than 2 others, and List with out size or capacity has lowest performance. Contiguous Memory Allocation : Contiguous memory allocation is basically a method in which a single contiguous section/part of memory is allocated to a process or file needing it.They do provide a dynamic, resizeable, and strongly-typed alternative to arrays. But, what makes Lists so important in C# Dynamic Size – Lists in C# have a dynamic size, meaning that you can add or remove elements as needed without having to worry about the size of the list.
Is list slower than array in C# : Relatively slower individual element access: Accessing individual elements in the List can be slower compared to an Array. It's not they're turtles, but Arrays are just cheetahs.