match() both are functions of re module in python. These functions are very efficient and fast for searching in strings. The function searches for some substring in a string and returns a match object if found, else it returns none.Structural pattern matching is a feature in some programming languages that allows you to compare objects based on their structure rather than just their type. This can be useful when you want to compare objects that may have different types but have the same underlying structure.The match function attempts to match a pattern against the beginning of the given string, as shown in Example 1-54. If the pattern matches anything at all (including an empty string, if the pattern allows that!), match returns a match object. The group method can be used to find out what matched.
What is the match function in Python Tutorialspoint : match() function returns a match object on success, None on failure. A match object instance contains information about the match: where it starts and ends, the substring it matched, etc. The match object's start() method returns the starting position of pattern in the string, and end() returns the endpoint.
Where is the match function
The MATCH Function[1] is categorized under Excel Lookup and Reference functions. It looks up a value in an array and returns the position of the value within the array.
How do you find matches in Python : Python offers different primitive operations based on regular expressions:
re. match() checks for a match only at the beginning of the string.
re.search() checks for a match anywhere in the string (this is what Perl does by default)
re. fullmatch() checks for entire string to be a match.
match(pattern, test_string) if result: print("Search successful.") else: print("Search unsuccessful.") Here, we used re. match() function to search pattern within the test_string . The method returns a match object if the search is successful. Therefore, pattern matching can be defined as: The process of searching for a specific sequence or placement of characters in a given set of data.
What is the difference between matching and searching in Python
match() checks for a match only at the beginning of the string. re.search() checks for a match anywhere in the string (this is what Perl does by default) re.A matching function is in general analogous to a production function. But whereas a production function usually represents the production of goods and services from inputs like labor and capital, a matching function represents the formation of new relationships from the pools of available unmatched individuals.The two formulas you can use to write the function are MATCH and XMATCH, which you can type as:=MATCH(lookup_value, lookup_array, [match_type])=XMATCH(lookup_value, lookup_array, [match_type])Where: "MATCH" is the function you want Excel to perform. Method 1: Using operators for Python string comparison
"==" (equal to),
"! =" (not equal to),
"<" (less than),
">" (greater than),
"<=" (less than or equal to), or.
">=" (greater than or equal to)
What is the difference between search and match in Python : search ⇒ find something anywhere in the string and return a match object. match ⇒ find something at the beginning of the string and return a match object.
How do you find matching words in a list in Python : Use the re.search() function to search for the string s in the list l If a match is found, print that the string s is present in the list If no match is found, print that the string s is not present in the list.
Why use pattern matching
You can use pattern matching to test the shape and values of the data instead of transforming it into a set of objects. The preceding example takes a string array, where each element is one field in the row. Pattern matching is the technique that identifies specific patterns or sequences or a combination of characters within a larger piece of information. Take an example of searching for a specific word in the book. You can use pattern matching to find all instances of that word present in the book.search ⇒ find something anywhere in the string and return a match object. match ⇒ find something at the beginning of the string and return a match object. match is much faster than search, so instead of doing regex.search("word") you can do regex.
What is the main difference between the match () method and the search () method : The match() method returns an array of matches. The search() method returns the position of the first match.
Antwort What is matching function in Python? Weitere Antworten – What is the match function in Python
match() both are functions of re module in python. These functions are very efficient and fast for searching in strings. The function searches for some substring in a string and returns a match object if found, else it returns none.Structural pattern matching is a feature in some programming languages that allows you to compare objects based on their structure rather than just their type. This can be useful when you want to compare objects that may have different types but have the same underlying structure.The match function attempts to match a pattern against the beginning of the given string, as shown in Example 1-54. If the pattern matches anything at all (including an empty string, if the pattern allows that!), match returns a match object. The group method can be used to find out what matched.
What is the match function in Python Tutorialspoint : match() function returns a match object on success, None on failure. A match object instance contains information about the match: where it starts and ends, the substring it matched, etc. The match object's start() method returns the starting position of pattern in the string, and end() returns the endpoint.
Where is the match function
The MATCH Function[1] is categorized under Excel Lookup and Reference functions. It looks up a value in an array and returns the position of the value within the array.
How do you find matches in Python : Python offers different primitive operations based on regular expressions:
match(pattern, test_string) if result: print("Search successful.") else: print("Search unsuccessful.") Here, we used re. match() function to search pattern within the test_string . The method returns a match object if the search is successful.
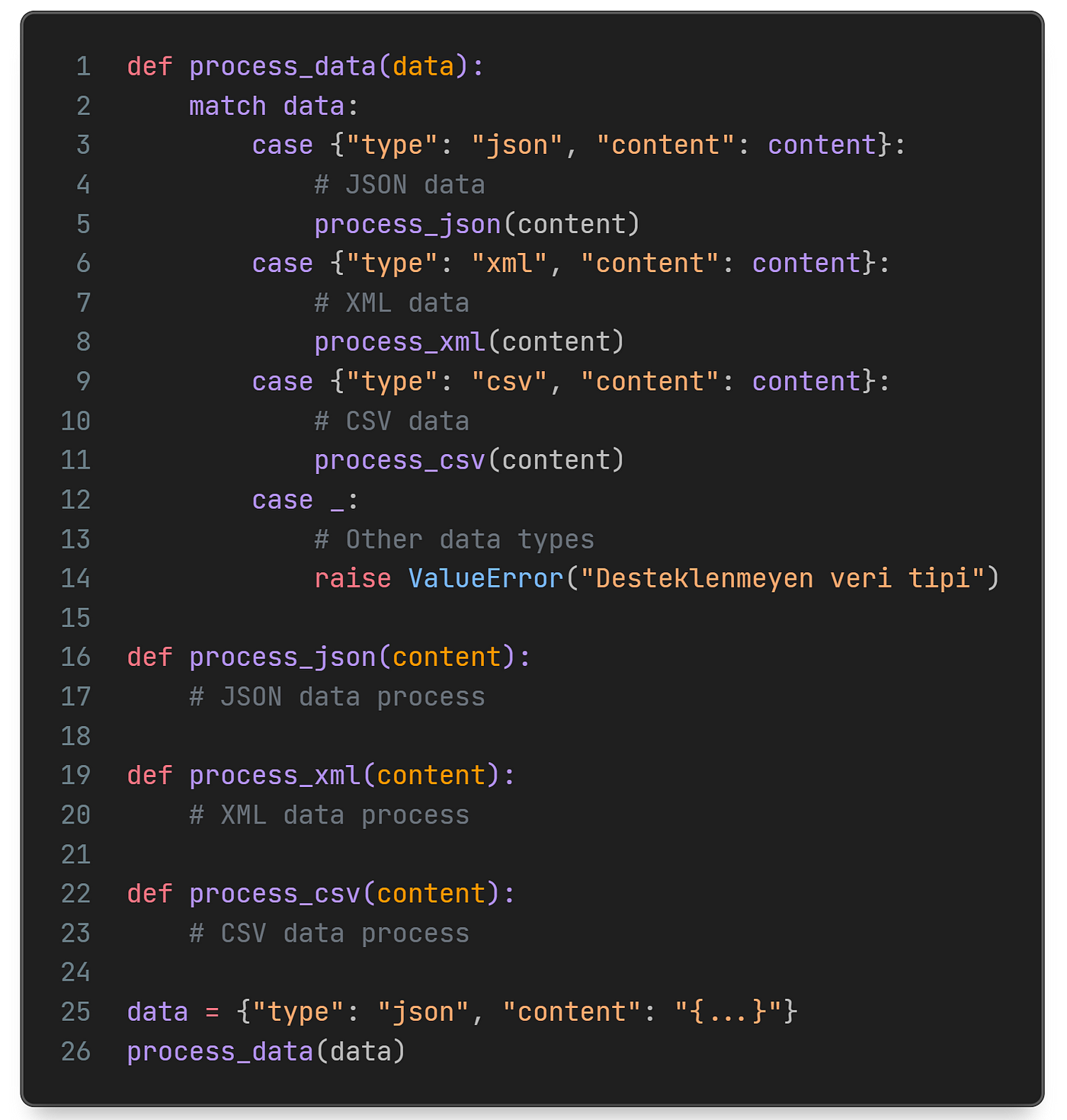
Therefore, pattern matching can be defined as: The process of searching for a specific sequence or placement of characters in a given set of data.
What is the difference between matching and searching in Python
match() checks for a match only at the beginning of the string. re.search() checks for a match anywhere in the string (this is what Perl does by default) re.A matching function is in general analogous to a production function. But whereas a production function usually represents the production of goods and services from inputs like labor and capital, a matching function represents the formation of new relationships from the pools of available unmatched individuals.The two formulas you can use to write the function are MATCH and XMATCH, which you can type as:=MATCH(lookup_value, lookup_array, [match_type])=XMATCH(lookup_value, lookup_array, [match_type])Where: "MATCH" is the function you want Excel to perform.
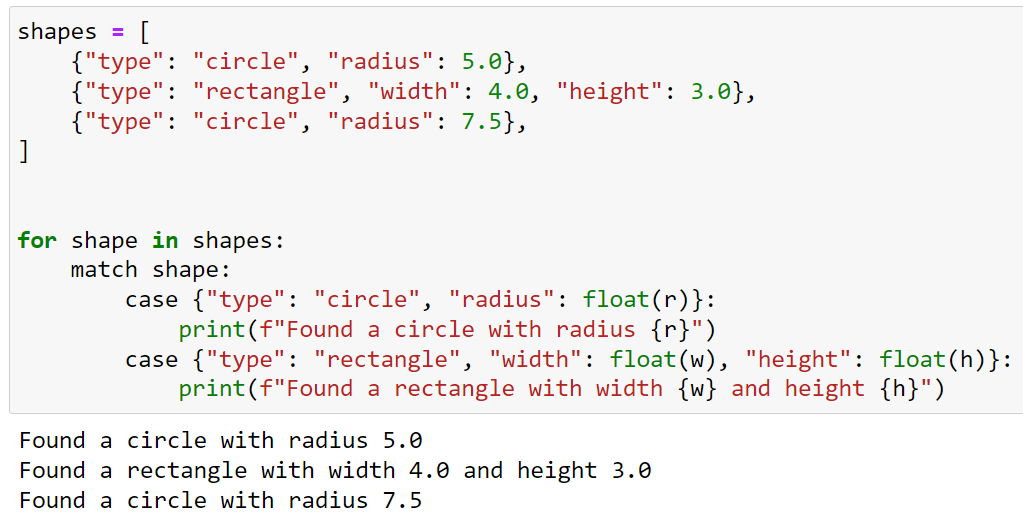
Method 1: Using operators for Python string comparison
What is the difference between search and match in Python : search ⇒ find something anywhere in the string and return a match object. match ⇒ find something at the beginning of the string and return a match object.
How do you find matching words in a list in Python : Use the re.search() function to search for the string s in the list l If a match is found, print that the string s is present in the list If no match is found, print that the string s is not present in the list.
Why use pattern matching
You can use pattern matching to test the shape and values of the data instead of transforming it into a set of objects. The preceding example takes a string array, where each element is one field in the row.
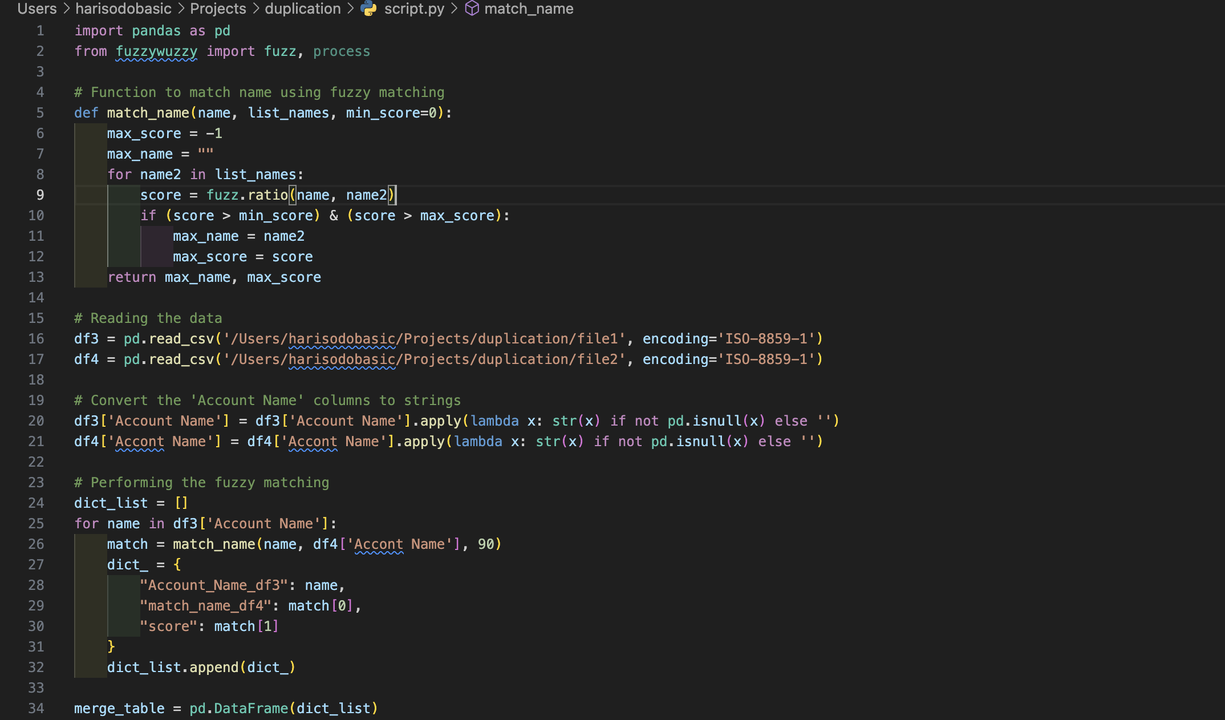
Pattern matching is the technique that identifies specific patterns or sequences or a combination of characters within a larger piece of information. Take an example of searching for a specific word in the book. You can use pattern matching to find all instances of that word present in the book.search ⇒ find something anywhere in the string and return a match object. match ⇒ find something at the beginning of the string and return a match object. match is much faster than search, so instead of doing regex.search("word") you can do regex.
What is the main difference between the match () method and the search () method : The match() method returns an array of matches. The search() method returns the position of the first match.