A hash code is a numeric value which is used to insert and identify an object in a hash-based collection. The GetHashCode method provides this hash code for algorithms that need quick checks of object equality.Hash codes are used to insert and retrieve keyed objects from hash tables efficiently. However, hash codes don't uniquely identify strings. Identical strings have equal hash codes, but the common language runtime can also assign the same hash code to different strings.In C#, when dealing with hash-based data structures like hash tables, dictionaries, and sets, we rely on two methods: GetHashCode() and Equals() . The GetHashCode() method creates a unique code for each item in these data structures, while the Equals() method is used to check if two objects are the same.
What is the hash code system : A hash function is used to quickly generate a number (hash code) that corresponds to the value of an object. Hash functions are usually specific to each type and, for uniqueness, must use at least one of the instance fields as input. Hash codes should not be computed by using the values of static fields.
Why use hash code
Hashing means using some function or algorithm to map object data to some representative integer value. This so-called hash code (or simply hash) can then be used as a way to narrow down our search when looking for the item in the map.
What is the role of hash code : Hash functions are used for data integrity and often in combination with digital signatures. With a good hash function, even a 1-bit change in a message will produce a different hash (on average, half of the bits change). With digital signatures, a message is hashed and then the hash itself is signed.
The hashCode() method returns the hash code of a string. where s[i] is the ith character of the string, n is the length of the string, and ^ indicates exponentiation.
If equals() returns true, then hashCodes of both objects have to be equal. If equals() returns false, then it is not required for hashCodes to be different for both objects, but it is reasonably practical and recommended. If hashCode() is different for objects, then equals() has to return false.
Why use hashCode and equals
In Java, the equals() and hashCode() methods are used to define object equality and to enable the effective use of objects in hash-based data structures such as HashMap, HashSet, etc. These methods are part of the Object class, which is the root class for all Java objects.Use of hashCode in Hashing
The hashcode value received is referred to as a bucket number. Keys with the same hash code are present in the same bucket. In the same bucket, multiple (key, value) pairs may be stored in the form of a linked list. However, keys with different hash codes reside in different buckets.For example, if the input is 123,456,789 and the hash table size 10,000, squaring the key produces 15,241,578,750,190,521, so the hash code is taken as the middle 4 digits of the 17-digit number (ignoring the high digit) 8750.
This can be accomplished with a quick application of a conditional operator, as seen below. public final class Boolean { private final boolean value; … public int hashCode() { return hashCode(value); } … public static int hashCode(boolean value) { return value 1231 : 1237; } … }
What is a hash and why is it important : Hashing is an important concept in cybersecurity as it allows for the safe encryption of data. This guide explains its principles and applications. Hashing is the practice of transforming a given key or string of characters into another value for the purpose of security.
Is hashCode faster than equals : Comparing two numbers is much faster than comparing two objects using the equals() method, especially if that method considers many fields. If our program compares objects, this is much simpler to do using a hash code.
Why do we need hashCode
Efficient Retrieval in Hash-Based Collections: Hash-based collections like HashMap , HashSet , and Hashtable use the hashCode() method to efficiently store and retrieve elements. It allows these data structures to quickly locate objects based on their hash code, resulting in faster access times.
Java String hashCode()
A hashcode is a number (object's memory address) generated from any object, not just strings. This number is used to store/retrieve objects quickly in a hashtable. Here, string is an object of the String class.Hashing helps to prevent file tampering or analyze file tampering by making it easy to identify each file. Since each file generates a hash value stored with the file data, any changes made to the file appear in the hash value.
What does a hash tell you : Hashes are a fundamental tool in computer security as they can reliably tell us when two files are identical, so long as we use secure hashing algorithms that avoid collisions.
Antwort What is a hash code in C#? Weitere Antworten – What is hash code in C#
A hash code is a numeric value which is used to insert and identify an object in a hash-based collection. The GetHashCode method provides this hash code for algorithms that need quick checks of object equality.Hash codes are used to insert and retrieve keyed objects from hash tables efficiently. However, hash codes don't uniquely identify strings. Identical strings have equal hash codes, but the common language runtime can also assign the same hash code to different strings.In C#, when dealing with hash-based data structures like hash tables, dictionaries, and sets, we rely on two methods: GetHashCode() and Equals() . The GetHashCode() method creates a unique code for each item in these data structures, while the Equals() method is used to check if two objects are the same.
What is the hash code system : A hash function is used to quickly generate a number (hash code) that corresponds to the value of an object. Hash functions are usually specific to each type and, for uniqueness, must use at least one of the instance fields as input. Hash codes should not be computed by using the values of static fields.
Why use hash code
Hashing means using some function or algorithm to map object data to some representative integer value. This so-called hash code (or simply hash) can then be used as a way to narrow down our search when looking for the item in the map.
What is the role of hash code : Hash functions are used for data integrity and often in combination with digital signatures. With a good hash function, even a 1-bit change in a message will produce a different hash (on average, half of the bits change). With digital signatures, a message is hashed and then the hash itself is signed.
The hashCode() method returns the hash code of a string. where s[i] is the ith character of the string, n is the length of the string, and ^ indicates exponentiation.
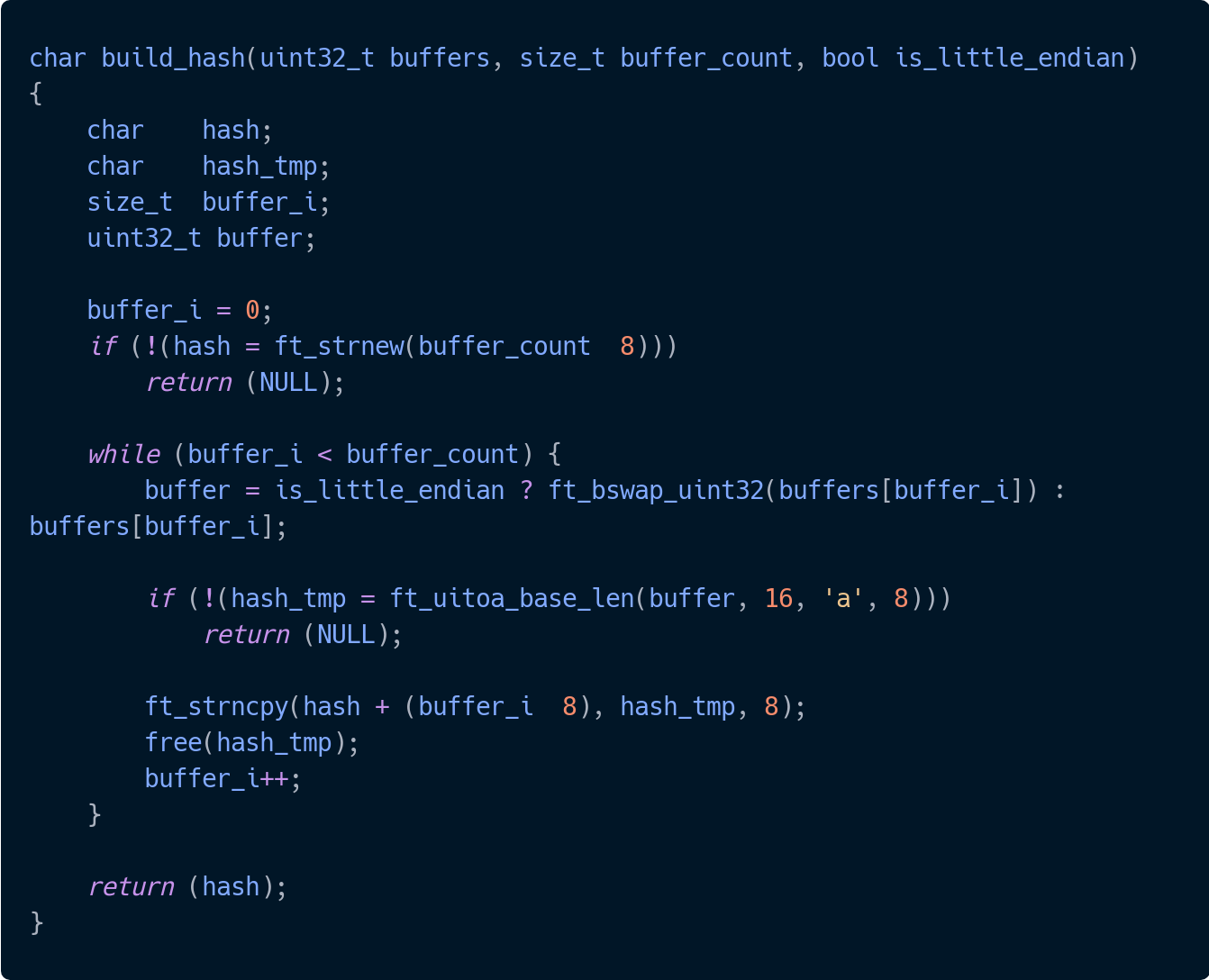
If equals() returns true, then hashCodes of both objects have to be equal. If equals() returns false, then it is not required for hashCodes to be different for both objects, but it is reasonably practical and recommended. If hashCode() is different for objects, then equals() has to return false.
Why use hashCode and equals
In Java, the equals() and hashCode() methods are used to define object equality and to enable the effective use of objects in hash-based data structures such as HashMap, HashSet, etc. These methods are part of the Object class, which is the root class for all Java objects.Use of hashCode in Hashing
The hashcode value received is referred to as a bucket number. Keys with the same hash code are present in the same bucket. In the same bucket, multiple (key, value) pairs may be stored in the form of a linked list. However, keys with different hash codes reside in different buckets.For example, if the input is 123,456,789 and the hash table size 10,000, squaring the key produces 15,241,578,750,190,521, so the hash code is taken as the middle 4 digits of the 17-digit number (ignoring the high digit) 8750.
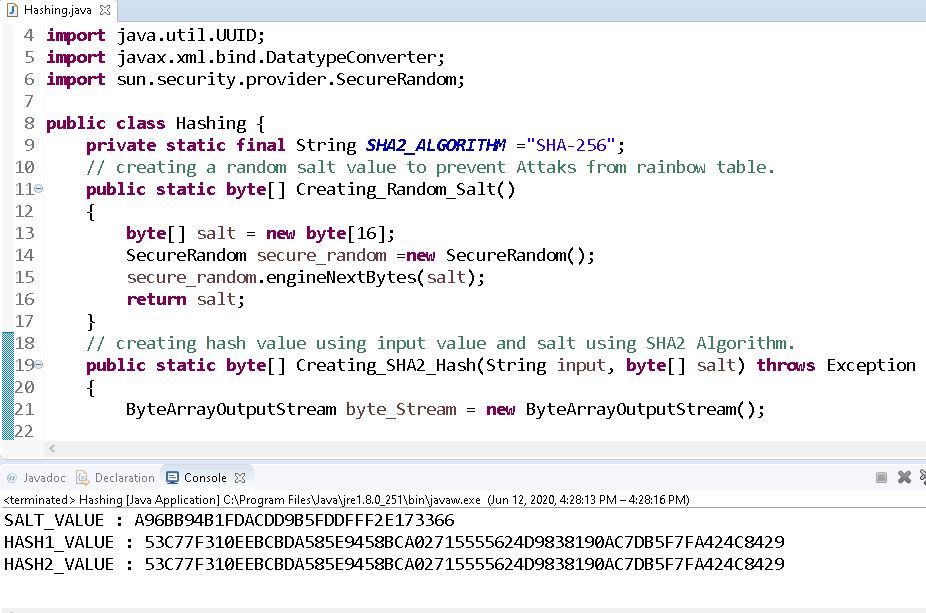
This can be accomplished with a quick application of a conditional operator, as seen below. public final class Boolean { private final boolean value; … public int hashCode() { return hashCode(value); } … public static int hashCode(boolean value) { return value 1231 : 1237; } … }
What is a hash and why is it important : Hashing is an important concept in cybersecurity as it allows for the safe encryption of data. This guide explains its principles and applications. Hashing is the practice of transforming a given key or string of characters into another value for the purpose of security.
Is hashCode faster than equals : Comparing two numbers is much faster than comparing two objects using the equals() method, especially if that method considers many fields. If our program compares objects, this is much simpler to do using a hash code.
Why do we need hashCode
Efficient Retrieval in Hash-Based Collections: Hash-based collections like HashMap , HashSet , and Hashtable use the hashCode() method to efficiently store and retrieve elements. It allows these data structures to quickly locate objects based on their hash code, resulting in faster access times.

Java String hashCode()
A hashcode is a number (object's memory address) generated from any object, not just strings. This number is used to store/retrieve objects quickly in a hashtable. Here, string is an object of the String class.Hashing helps to prevent file tampering or analyze file tampering by making it easy to identify each file. Since each file generates a hash value stored with the file data, any changes made to the file appear in the hash value.
What does a hash tell you : Hashes are a fundamental tool in computer security as they can reliably tell us when two files are identical, so long as we use secure hashing algorithms that avoid collisions.