This can be accomplished with a quick application of a conditional operator, as seen below. public final class Boolean { private final boolean value; … public int hashCode() { return hashCode(value); } … public static int hashCode(boolean value) { return value 1231 : 1237; } … }hashCode() method of String class can be used to convert a string into hash code. hashCode() method will return either negative or positive integer hash values. The returned hash value cannot be re-converted back to string once hashed.How to create hash from string in JavaScript
Using JavaScript charCodeAt() method.
Using crypto.createHash() method.
Using JavaScript String's reduce() Method.
Using bitwise XOR operation.
How to get the hashCode of a string in Java : Java String hashCode() Method
The hashCode() method returns the hash code of a string. where s[i] is the ith character of the string, n is the length of the string, and ^ indicates exponentiation.
How to generate sha256 code
How to use the SHA-256 hash generator
Type in the text to be hashed in the input box.
Click the "Generate" button.
Get your SHA-256 hash in the output box.
Click on the output box to get copy the code to your clipboard.
Is MD5 still used : As of 2019, MD5 continues to be widely used, despite its well-documented weaknesses and deprecation by security experts. A collision attack exists that can find collisions within seconds on a computer with a 2.6 GHz Pentium 4 processor (complexity of 224.1).
Hashing: This is a one-way process that converts data into a fixed-length alphanumeric string. A hashed string is sometimes called a hash. Hashes are often used to verify the integrity of data. When two identical strings are hashed with the same algorithm, their hashes are the same. String hashing is the technique or process of mapping string and integer. In other words, String hashing is the way to convert the string into an integer, and that integer is known as the hash of a string. String hashing is used to compare two strings whether they are equal or not.
How to generate sha256 in JavaScript
To generate a SHA-256 hash in Node.js using crypto:
To set the key/value pair in your Hash Table, you need to write a set() method that accepts (key, value) as its parameters: The set() method will call the _hash() method to get the index value. The [key, value] pair will be assigned to the table at the specified index. Then, the size property will be incremented by one.Example 1
public class IntegerHashCodeExample1 {
public static void main(String[] args)
{
//Create integer object.
Integer i = new Integer("155");
//Returned hash code value for this object.
int hashValue = i.hashCode();
System.out.println("Hash code Value for object is: " + hashValue);
The hashCode() uses an internal hash function that returns the hash value of the stored value in the String variable. Hash Value: This is the encrypted value that is generated with the help of some hash function. For example, the hash value of 'A' is 67.
Is SHA-256 still secure : Many consider SHA-256 to be one of the most secure hashing algorithms today. This is because it's great at preventing values from being reversed back to the original content. Another problem that it solves well is avoiding hashing collisions. This means that two separate inputs cannot produce an identical hash.
How to generate SHA-256 hash in cmd : SHA-256 checksum tool is called sha256sum.
Go to the directory where your downloaded file is stored, e.g.: cd home/downloads.
Use the following command to generate the checksum: sha256sum my_file.exe.
Compare the generated value to the checksum of the file in Rublon Downloads.
Is MD5 better than SHA-256
SHA256 has several advantages over MD5 and SHA-1, such as producing a longer hash (256 bits) that is more resistant to collisions and brute-force attacks. Additionally, there are no known vulnerabilities or weaknesses with SHA256, unlike MD5 and SHA-1 which have been exploited by hackers and researchers. Vulnerabilities: The MD5 algorithm has long been considered insecure for cryptographic purposes due to significant vulnerabilities. Researchers have demonstrated practical collision attacks against MD5, which allows for the creation of different inputs that produce the same hash value.Hash codes are used to insert and retrieve keyed objects from hash tables efficiently. However, hash codes don't uniquely identify strings. Identical strings have equal hash codes, but the common language runtime can also assign the same hash code to different strings.
Is hashing an encoding : Encoding is a technique where the data is transformed from one form to another. Hashing is a technique where the data is converted to hash using different algorithms present there. Encryption is a technique used for protecting the confidentiality of the data. Encryption is used for preserving the usability of the data.
Antwort How to create hash code? Weitere Antworten – How to generate hash code
This can be accomplished with a quick application of a conditional operator, as seen below. public final class Boolean { private final boolean value; … public int hashCode() { return hashCode(value); } … public static int hashCode(boolean value) { return value 1231 : 1237; } … }hashCode() method of String class can be used to convert a string into hash code. hashCode() method will return either negative or positive integer hash values. The returned hash value cannot be re-converted back to string once hashed.How to create hash from string in JavaScript
How to get the hashCode of a string in Java : Java String hashCode() Method
The hashCode() method returns the hash code of a string. where s[i] is the ith character of the string, n is the length of the string, and ^ indicates exponentiation.
How to generate sha256 code
How to use the SHA-256 hash generator
Is MD5 still used : As of 2019, MD5 continues to be widely used, despite its well-documented weaknesses and deprecation by security experts. A collision attack exists that can find collisions within seconds on a computer with a 2.6 GHz Pentium 4 processor (complexity of 224.1).
Hashing: This is a one-way process that converts data into a fixed-length alphanumeric string. A hashed string is sometimes called a hash. Hashes are often used to verify the integrity of data. When two identical strings are hashed with the same algorithm, their hashes are the same.
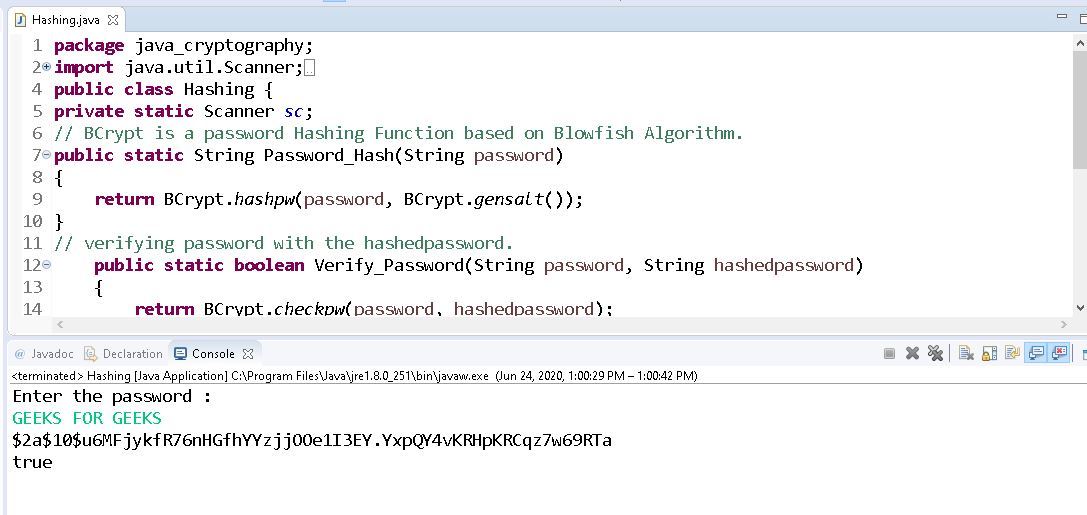
String hashing is the technique or process of mapping string and integer. In other words, String hashing is the way to convert the string into an integer, and that integer is known as the hash of a string. String hashing is used to compare two strings whether they are equal or not.
How to generate sha256 in JavaScript
To generate a SHA-256 hash in Node.js using crypto:
To set the key/value pair in your Hash Table, you need to write a set() method that accepts (key, value) as its parameters: The set() method will call the _hash() method to get the index value. The [key, value] pair will be assigned to the table at the specified index. Then, the size property will be incremented by one.Example 1
The hashCode() uses an internal hash function that returns the hash value of the stored value in the String variable. Hash Value: This is the encrypted value that is generated with the help of some hash function. For example, the hash value of 'A' is 67.
Is SHA-256 still secure : Many consider SHA-256 to be one of the most secure hashing algorithms today. This is because it's great at preventing values from being reversed back to the original content. Another problem that it solves well is avoiding hashing collisions. This means that two separate inputs cannot produce an identical hash.
How to generate SHA-256 hash in cmd : SHA-256 checksum tool is called sha256sum.
Is MD5 better than SHA-256
SHA256 has several advantages over MD5 and SHA-1, such as producing a longer hash (256 bits) that is more resistant to collisions and brute-force attacks. Additionally, there are no known vulnerabilities or weaknesses with SHA256, unlike MD5 and SHA-1 which have been exploited by hackers and researchers.

Vulnerabilities: The MD5 algorithm has long been considered insecure for cryptographic purposes due to significant vulnerabilities. Researchers have demonstrated practical collision attacks against MD5, which allows for the creation of different inputs that produce the same hash value.Hash codes are used to insert and retrieve keyed objects from hash tables efficiently. However, hash codes don't uniquely identify strings. Identical strings have equal hash codes, but the common language runtime can also assign the same hash code to different strings.
Is hashing an encoding : Encoding is a technique where the data is transformed from one form to another. Hashing is a technique where the data is converted to hash using different algorithms present there. Encryption is a technique used for protecting the confidentiality of the data. Encryption is used for preserving the usability of the data.