RegExp.prototype.test() The test() method of RegExp instances executes a search with this regular expression for a match between a regular expression and a specified string. Returns true if there is a match; false otherwise.How to read (and write) regexes
a|b – Match either “a” or “b.
– Zero or one.
+ – one or more.
* – zero or more.
{N} – Exactly N number of times (where N is a number)
{N,} – N or more number of times (where N is a number)
{N,M} – Between N and M number of times (where N and M are numbers and N < M)
*
A regex pattern matches a target string. The pattern is composed of a sequence of atoms. An atom is a single point within the regex pattern which it tries to match to the target string. The simplest atom is a literal, but grouping parts of the pattern to match an atom will require using ( ) as metacharacters.
How to get matched string from regex : If you need to know if a string matches a regular expression RegExp , use RegExp.prototype.test() . If you only want the first match found, you might want to use RegExp.prototype.exec() instead.
How to check if regex is valid or not
The static Regex. Match method returns a single Match object. By using this static method to run a regular expression against a string (in this case a blank string), we can determine whether the regular expression is invalid by watching for a thrown exception.
How to test regex pattern : How to do Python Regex Testing
re. match(): Checks if the pattern matches at the beginning of the string.
re.search(): Searches the entire string for a match.
re. findall(): Finds all occurrences of the pattern in the string.
re. finditer(): Returns an iterator of match objects for all occurrences.
re.
compile() (used to compile regex patterns) function within the try block. This will firstly try to compile the pattern and if any exception occurs during the compilation, it would firstly check whether it is re. error, if it is then only the except block will execute. *$ means – match, from beginning to end, any character that appears zero or more times. Basically, that means – match everything from start to end of the string. This regex pattern is not very useful.
Is regex matching fast
Regular expression matching can be simple and fast, using finite automata-based techniques that have been known for decades.The Match(String) method returns the first substring that matches a regular expression pattern in an input string. For information about the language elements used to build a regular expression pattern, see Regular Expression Language – Quick Reference.IsMatch(String, String) Indicates whether the specified regular expression finds a match in the specified input string. When you create a text question, along with word/character limits, you can validate for Regex pattern matching. To validate a field with a Regex pattern, click the Must match pattern check box. Next, add the expression you want to validate against. Then add the message your users will see if the validation fails.
How do you validate a pattern in regex : To validate a field with a Regex pattern, click the Must match pattern check box. Next, add the expression you want to validate against. Then add the message your users will see if the validation fails.
How to validate the regular expression : The Validation (Regex) property helps you define a set of validation options for a given field. In general, this field property is used to perform validation checks (format, length, etc.) on the value that the user enters in a field. If the user enters a value that does not pass these checks, it will throw an error.
What is .*) in regex
(. *) matches any character ( . ) any number of times ( * ), as few times as possible to make the regex match ( ). The Match-one-or-more Operator ( + or \+ )
This operator is similar to the match-zero-or-more operator except that it repeats the preceding regular expression at least once; see section The Match-zero-or-more Operator ( * ), for what it operates on, how some syntax bits affect it, and how Regex backtracks to match it.Despite being hard to read, hard to validate, hard to document and notoriously hard to master, regexes are still widely used today. Supported by all modern programming languages, text processing programs and advanced text editors, regexes are now used in more than a third of both Python and JavaScript projects.
Why is regex expensive : regex is expensive – regex is often the most CPU-intensive part of a program. And a non-matching regex can be even more expensive to check than a matching one. regex is greedy – It's extremely easy to match much more than intended, leading to bugs.
Antwort How do I know if regex matches? Weitere Antworten – How do you check if regex is a match
RegExp.prototype.test() The test() method of RegExp instances executes a search with this regular expression for a match between a regular expression and a specified string. Returns true if there is a match; false otherwise.How to read (and write) regexes
A regex pattern matches a target string. The pattern is composed of a sequence of atoms. An atom is a single point within the regex pattern which it tries to match to the target string. The simplest atom is a literal, but grouping parts of the pattern to match an atom will require using ( ) as metacharacters.
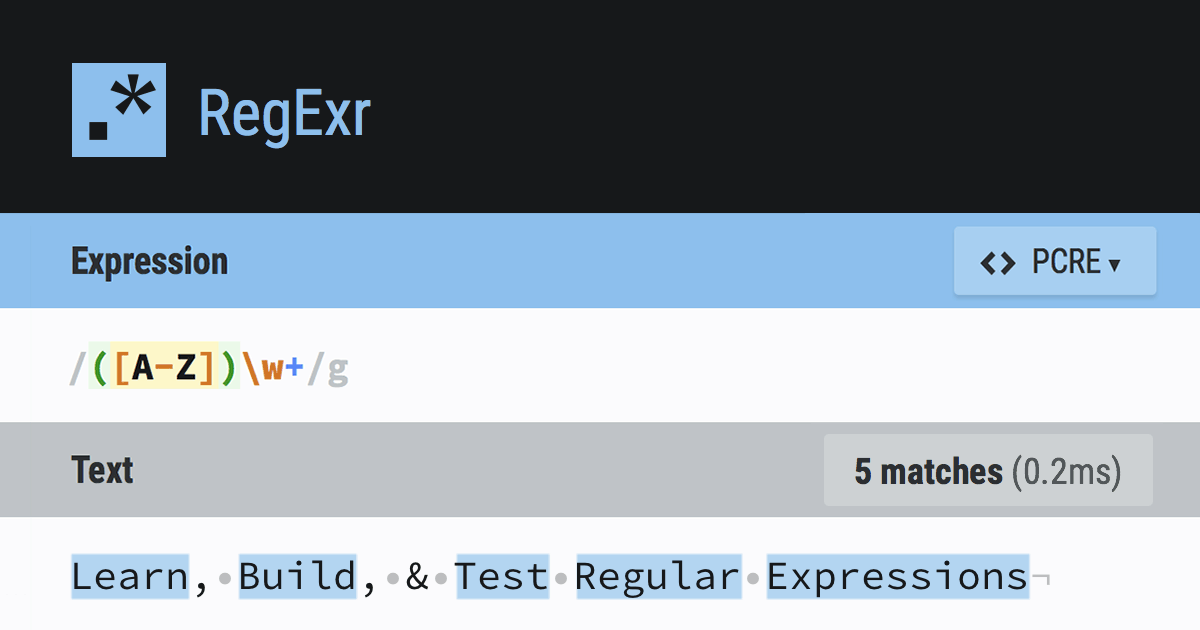
How to get matched string from regex : If you need to know if a string matches a regular expression RegExp , use RegExp.prototype.test() . If you only want the first match found, you might want to use RegExp.prototype.exec() instead.
How to check if regex is valid or not
The static Regex. Match method returns a single Match object. By using this static method to run a regular expression against a string (in this case a blank string), we can determine whether the regular expression is invalid by watching for a thrown exception.
How to test regex pattern : How to do Python Regex Testing
compile() (used to compile regex patterns) function within the try block. This will firstly try to compile the pattern and if any exception occurs during the compilation, it would firstly check whether it is re. error, if it is then only the except block will execute.
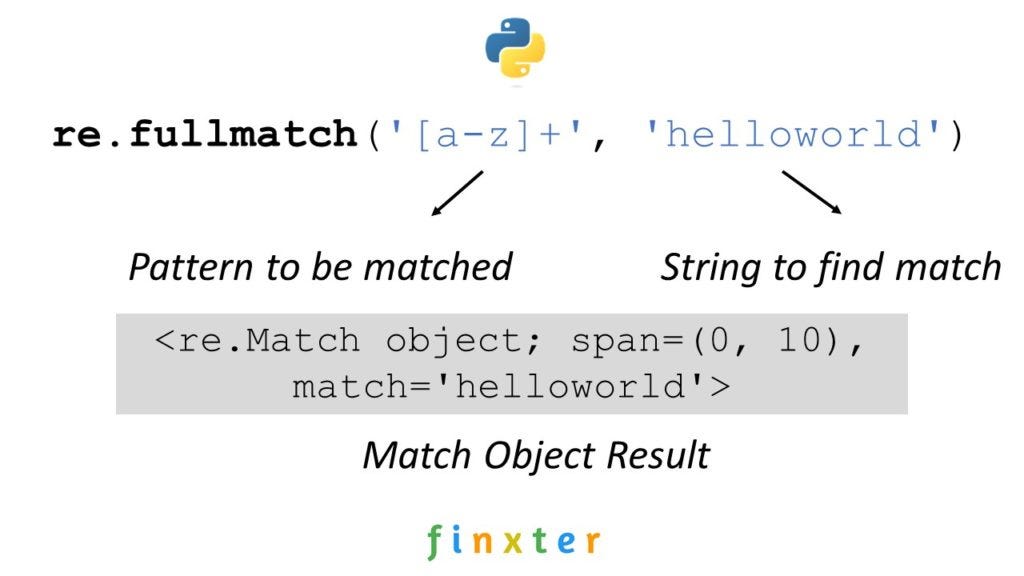
*$ means – match, from beginning to end, any character that appears zero or more times. Basically, that means – match everything from start to end of the string. This regex pattern is not very useful.
Is regex matching fast
Regular expression matching can be simple and fast, using finite automata-based techniques that have been known for decades.The Match(String) method returns the first substring that matches a regular expression pattern in an input string. For information about the language elements used to build a regular expression pattern, see Regular Expression Language – Quick Reference.IsMatch(String, String) Indicates whether the specified regular expression finds a match in the specified input string.

When you create a text question, along with word/character limits, you can validate for Regex pattern matching. To validate a field with a Regex pattern, click the Must match pattern check box. Next, add the expression you want to validate against. Then add the message your users will see if the validation fails.
How do you validate a pattern in regex : To validate a field with a Regex pattern, click the Must match pattern check box. Next, add the expression you want to validate against. Then add the message your users will see if the validation fails.
How to validate the regular expression : The Validation (Regex) property helps you define a set of validation options for a given field. In general, this field property is used to perform validation checks (format, length, etc.) on the value that the user enters in a field. If the user enters a value that does not pass these checks, it will throw an error.
What is .*) in regex
(. *) matches any character ( . ) any number of times ( * ), as few times as possible to make the regex match ( ).
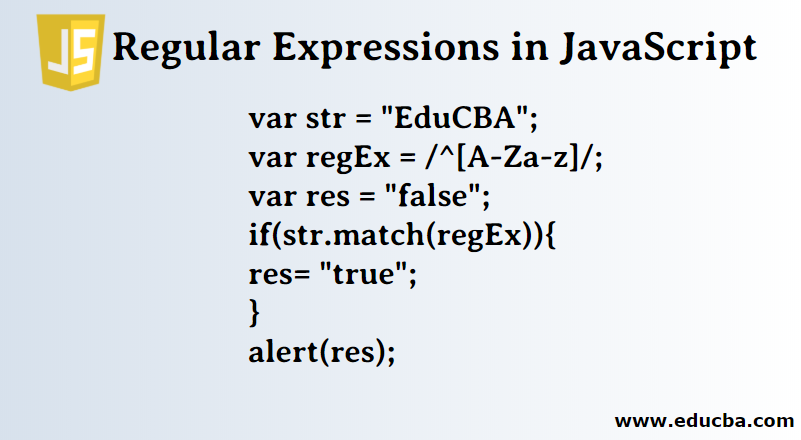
The Match-one-or-more Operator ( + or \+ )
This operator is similar to the match-zero-or-more operator except that it repeats the preceding regular expression at least once; see section The Match-zero-or-more Operator ( * ), for what it operates on, how some syntax bits affect it, and how Regex backtracks to match it.Despite being hard to read, hard to validate, hard to document and notoriously hard to master, regexes are still widely used today. Supported by all modern programming languages, text processing programs and advanced text editors, regexes are now used in more than a third of both Python and JavaScript projects.
Why is regex expensive : regex is expensive – regex is often the most CPU-intensive part of a program. And a non-matching regex can be even more expensive to check than a matching one. regex is greedy – It's extremely easy to match much more than intended, leading to bugs.